Managed Global SQL
Seaplane comes with a built-in managed SQL database to build powerful data science and machine learning applications. The Seaplane SDK makes connecting with and using your DB as simple as possible by handing you a single authenticated SQL object to work with inside your tasks.
Database Creation​
You can create a new database on the
Flightdeck. In the resources tab select type
SQL DB
, select your project and click create.

The Flightdeck shows your credentials once and does not store them. Make
sure you save them in a secure place. You can download them as a .txt
or
.json
file.
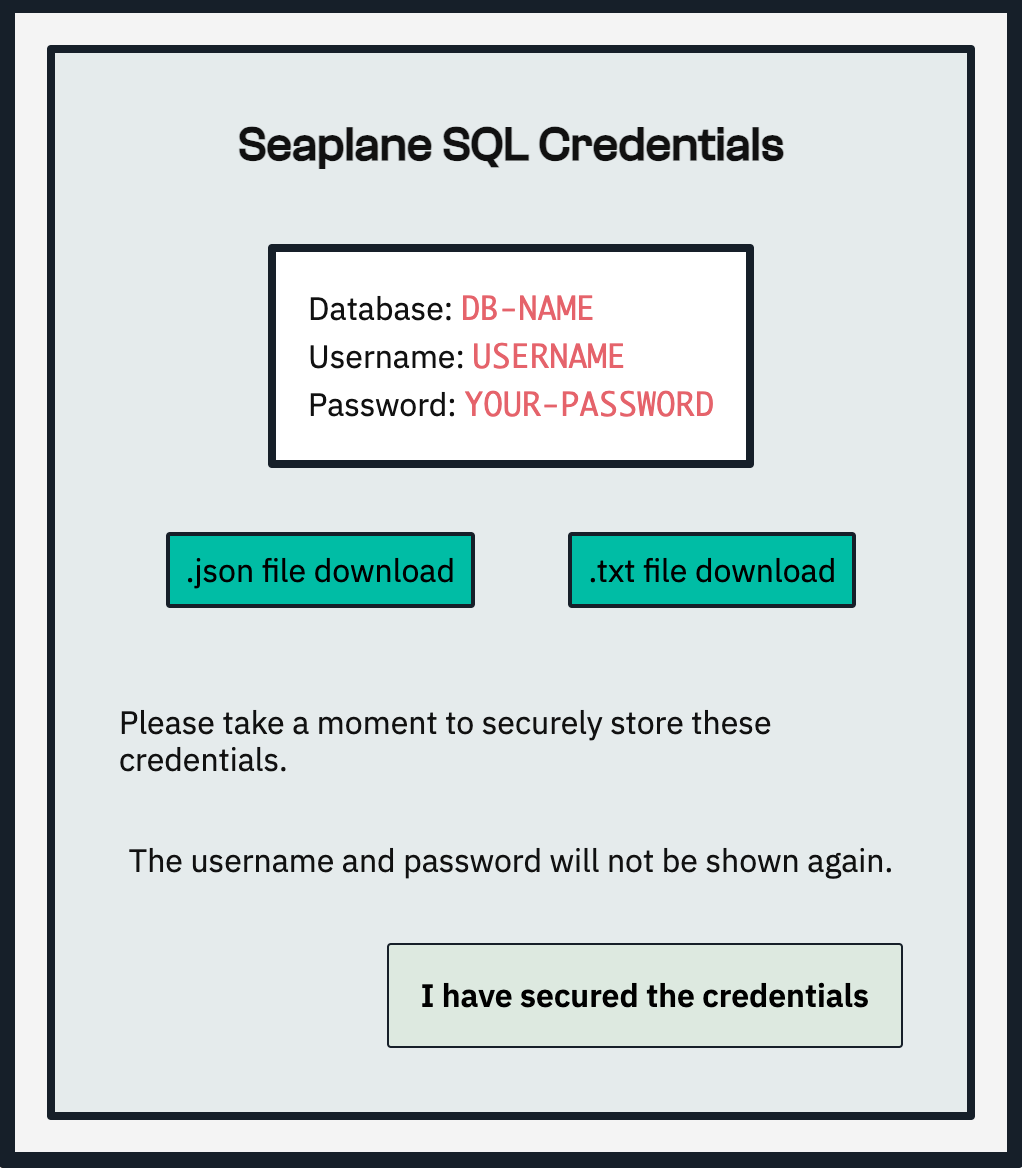
Authentication​
Create a sql_access
object to use your database in a task. For security
reasons, we recommend you store the login credentials in the Seaplane Secrets
Store and load them as environment
variables.
Add the SQL access object to any @task
as follows to give it database access.
Seaplane handles all the complexity of connecting to the database, creating
cursors, executing queries and everything else to use the database.
from seaplane import task
import os
# create a SQL access object
sql_access = {
"username": os.getenv('db_username'),
"password": os.getenv('db_password'),
"database": os.getenv('db_name')
}
@task(id='my-sql-task', sql=sql_access)
def my_sql_task(data, sql):
# your task code here
Querying The Database​
You can query the database by calling the SQL object with the execute
method.
The execute method supports any legal SQL query supported by Postgress 11.
For example, to insert a query into a table you run the following code.
from seaplane import task
import os
# create a SQL access object
sql_access = {
"username": os.getenv('db_username'),
"password": os.getenv('db_password'),
"database": os.getenv('db_name')
}
@task(id='my-sql-task', sql=sql_access)
def my_sql_task(data, sql):
# run SQL queries
sql.execute("INSERT INTO my_table ....")
Geofencing And Global Features​
We are soon extending Seaplane Managed SQL with global geofencing features. Geofencing allows users to restrict workloads to specific regions or countries enabling data regulation-compliant applications.
Coming soon! Reach out if you want early access via support@seaplane.io